自定义组件的记录和数组属性
最受期待的功能之一终于上线了:用于自定义TypeScript和JavaScript组件的记录和数组属性类型。
我们迫不及待地想看看利用它们能创造出多么惊人的体验!
记录
现在可以使用record
属性将属性分组到一个JavaScript类中:
1import {Component, Property} from '@wonderlandengine/api';
2
3class PlayerSettings {
4 static Properties = {
5 speed: Property.float(10.0),
6 name: Property.string('Hero')
7 };
8}
9
10export class PlayerManager extends Component {
11 static TypeName = 'player-manager';
12
13 static Properties = {
14 settings: Property.record(PlayerSettings)
15 };
16
17 start() {
18 console.log(this.settings); // 输出 Player(10.0, 'Hero')
19 console.log(this.settings instanceof PlayerSettings); // true
20 }
21}
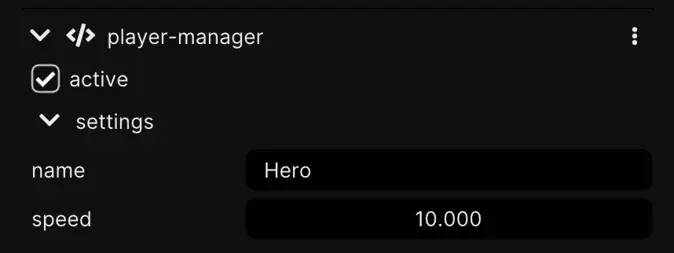
请注意定义PlayerSettings
类的风格。属性的声明方式对于记录和组件是相同的。
数组
现在也可以使用array
属性创建属性列表:
Property.string()
表示数组元素的类型。数组一次只能包含单一类型的元素。
结合record
属性后,现在可以实现复杂类型:
1import {Component, Property} from '@wonderlandengine/api';
2
3class Zombie {
4 static Properties = {
5 name: Property.string(),
6 maxHealth: Property.float(100.0),
7 mesh: Property.mesh(),
8 };
9}
10
11export class ZombieSpawner extends Component {
12 static TypeName = 'zombie-spawner';
13
14 static Properties = {
15 enemies: Property.array(Property.record(Zombie))
16 };
17
18 start() {
19 for(const zombie of this.enemies) {
20 const name = zombie.name;
21 const health = zombie.maxHealth;
22 // 生成这只可怕的僵尸!
23 }
24 }
25}
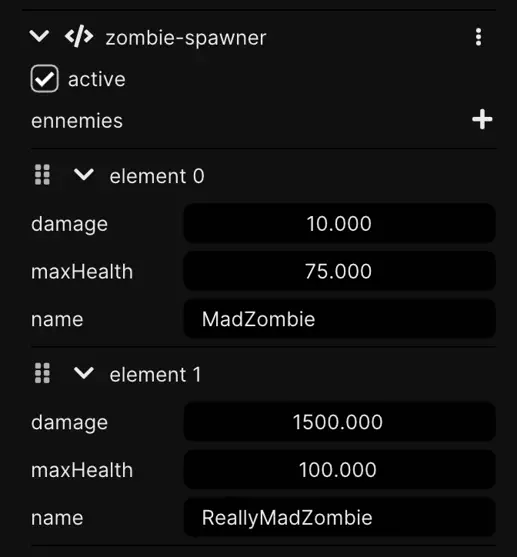
该API还支持嵌套数组属性(数组的数组):
1import {Component, Property} from '@wonderlandengine/api';
2
3export class LevelGenerator extends Component {
4 static TypeName = 'level-generator';
5
6 static Properties = {
7 mapPiecesPerLevel: Property.array(Property.array(Property.string())),
8 };
9
10 start() {
11 // 输出类似:
12 // [
13 // ['level_1_forest.map', 'level_1_mountains.map'],
14 // ['level_2_beach.map', 'level_2_ocean.map', 'level_2_desert.map'],
15 // ['level_3_underwater.map'],
16 // ]
17 console.log(this.mapPiecesPerLevel);
18 }
19}
TypeScript
对于TypeScript用户,全新的record
和array
属性装饰器使得声明复杂的组件属性更加紧凑和直观:
1import {Component, property, Property}from '@wonderlandengine/api';
2
3class Zombie {
4 @property.string()
5 name!: string;
6
7 @property.float(100)
8 maxHealth!: number;
9
10 @property.float(10)
11 damage!: number;
12}
13
14export class ZombieManager extends Component {
15 static TypeName = 'zombie-manager';
16
17 @property.array(Property.record(Zombie))
18 zombies!: Zombie[];
19}
Last Update: May 12, 2025